Angular Framework Introduction
.......................................................................................................................................................................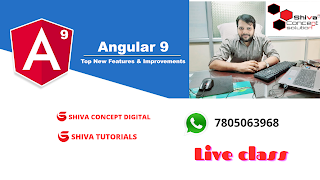
before study this first you learn JS and Jquery:-
https://www.shivatutorials.com/search/label/JAVASCRIPT
https://www.shivatutorials.com/search/label/Jquery
It is a javascript framework which provides an MVC design pattern to develop dynamic web application using HTML and JavaScript Object.
MVC means Model, View, and Controller, it is used to distribute the project on different project layer.
Angular JS-Support JavaScript code and Typescript code. structure to write Business Code.
The flow of MVC:-
MVC flow is the opposite of there name.
The First Controller module will be loaded then View will be loaded after that model object will be integrated.
Controller
View Model
Controller:- It is used to provide routing and business code of an application using Javascript.
we will write JavaScript code to create a controller
View:- HTML web page will work to implement View,it will provide a dynamic User interface to bind Html data to the model object.
Model:- it contains data of the application and shows into view.
Angular provides an angular plugin which contains angular libraries to use angular functionality.
Difference between Angular Js and Angular:-
Angular Js is the initial version of angular it is also called angular 1.0.
It uses Javascript to write code and it is fully dependent on HTML documents.
It provides MVC design patterns to develop dynamic applications but it is not compatible with mobile application development.
hence Angular Js 2.0,4.0,5.0,6.0,7.0 and 8.0 , 17 version has been released which uses TypeScript (Object-Oriented JavaScript ) to write code and it is completely depend on Module and Component-based architecture.
MVC means Model, View, and Controller, it is used to distribute the project on different project layer.
Angular JS-Support JavaScript code and Typescript code. structure to write Business Code.
The flow of MVC:-
MVC flow is the opposite of there name.
The First Controller module will be loaded then View will be loaded after that model object will be integrated.
Controller
View Model
Controller:- It is used to provide routing and business code of an application using Javascript.
we will write JavaScript code to create a controller
View:- HTML web page will work to implement View,it will provide a dynamic User interface to bind Html data to the model object.
Model:- it contains data of the application and shows into view.
Angular provides an angular plugin which contains angular libraries to use angular functionality.
Difference between Angular Js and Angular:-
Angular Js is the initial version of angular it is also called angular 1.0.
It uses Javascript to write code and it is fully dependent on HTML documents.
It provides MVC design patterns to develop dynamic applications but it is not compatible with mobile application development.
hence Angular Js 2.0,4.0,5.0,6.0,7.0 and 8.0 , 17 version has been released which uses TypeScript (Object-Oriented JavaScript ) to write code and it is completely depend on Module and Component-based architecture.
How to create App in Angular without state.
Step 1st:
ng new myangproject --no-standalone
Step2nd:-
Create Controller
ng g c addition
Step3rd:-
import FormsModule under app.module.ts file
update file after adding FormsModule
import { NgModule } from '@angular/core';
import { BrowserModule, provideClientHydration } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { AdditionComponent } from './addition/addition.component';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [
AppComponent,
AdditionComponent
],
imports: [
FormsModule,
BrowserModule,
AppRoutingModule
],
providers: [
provideClientHydration()
],
bootstrap: [AppComponent]
})
export class AppModule { }
Step4th:
Create Addition form designing under angular component design file
<p>addition works!</p>
<input type="number" [(ngModel)]="a" placeholder="Enter First Number" />
<br/><br/>
<input type="number" [(ngModel)]="b" placeholder="Enter Second Number" />
<br/><br/>
<input type="button" value="Addition" (click)="add()" />
<br/>
{{c}}
Step5th:-
import { Component } from '@angular/core';
@Component({
selector: 'app-addition',
templateUrl: './addition.component.html',
styleUrl: './addition.component.css'
})
export class AdditionComponent {
a:number=12
b:number=2
c:number=0
add():void {
this.c = this.a+this.b;
}
}
2) Create Another Example of Angular to implement Addition, Subtraction, Multiplication and Division
using four separate button.
Create Component and Add following code on Html file:
<p>addition works!</p>
<input type="number" [(ngModel)]="a" placeholder="Enter First Number" />
<br/><br/>
<input type="number" [(ngModel)]="b" placeholder="Enter Second Number" />
<br/><br/>
<input type="button" value="Addition" (click)="add($event)" />
<br/>
<input type="button" value="Substraction" (click)="add($event)" />
<br/>
<input type="button" value="Multiplication" (click)="add($event)" />
<br/>
<input type="button" value="Division" (click)="add($event)" />
{{c}}
Write following code under typescript file
import { Component } from '@angular/core';
@Component({
selector: 'app-addition',
templateUrl: './addition.component.html',
styleUrl: './addition.component.css'
})
export class AdditionComponent {
a:number=12
b:number=2
c:number=0
param=null;
add(event:any):void {
this.param = event.target.value;
if(this.param=="Addition")
{
this.c = this.a+this.b;
}
else if(this.param=="Substraction")
{
this.c = this.a-this.b;
}
else if(this.param=="Multiplication")
{
this.c = this.a*this.b;
}
else if(this.param=="Division")
{
this.c = this.a/this.b;
}
alert("hello"+this.a);
}
}
3) How to bind data in dropdownlist
Declare array in typescript file
courses = ['C', 'C++','DS', 'JAVA'];
Display data under html file
<select >
<option *ngFor="let c of courses" [value]="c">{{c}}</option>
</select>
<br/>
<select multiple>
<option *ngFor="let c of courses" [value]="c">{{c}}</option>
</select>
Create Angular Form using Radiobutton, Checkbox, Dropdownlist and Listbox:-
Code of Design File:-
<p>myform works!</p>
<hr/>
<select [(ngModel)]="course">
<option value="">Select Course </option>
<option *ngFor="let c of courses" [value]="c">{{c}}</option>
</select>
<br/><br/>
<p>Select Course</p>
<select [(ngModel)]="course1" multiple>
<option *ngFor="let c of courses" [value]="c">{{c}}</option>
</select>
<br/><br/>
<input type="radio" value="JAVA" name="basic" [(ngModel)]="advance"/>Java<br/>
<input type="radio" value=".NET" name="basic" [(ngModel)]="advance"/>.NET <br/>
<br/><br/>
<input type="checkbox" value="C" name="basic1" [(ngModel)]="basic1"/>C <br/>
<input type="checkbox" value="CPP" name="basic2" [(ngModel)]="basic2"/>CPP <br/>
<input type="button" value="Submit" (click)="displayData()" />
<br>
{{result}}
Code of Javascript:-
import { Component } from '@angular/core';
@Component({
selector: 'app-myform',
templateUrl: './myform.component.html',
styleUrl: './myform.component.css'
})
export class MyformComponent {
courses = ['C', 'C++','DS', 'JAVA'];
course='';
course1='';
result = '';
basic1 = '';
basic2 = '';
advance='';
displayData():void
{
var arr= this.course1;
var data='';
var s1='',s2='';
for(var i=0;i<arr.length;i++)
{
data = data + arr[i] + " ";
}
if(this.basic1)
{
s1=" C ";
}
else
{
s1 = " ";
}
if(this.basic2)
{
s2 = " CPP ";
}
else{
s2 = '';
}
this.result = "Selected Course is "+this.course + " Other courses "+data + " Basic Course is "+ (s1 + s2) + " Advance course is "+this.advance;
// alert("Selected Course is "+this.course + " Other courses "+data);
}
}
How to pass parameter to one component to another:-
1) First create path
2) create First Component:-
import { Router } from '@angular/router';
submitData3()
{
var id = 10
this.router.navigate(['/addition',id]);
}
Code of Design File
<input type="button" value="Addition Component" (click)="submitData3()" />
3) Create Addition Component to get the data from Url:-
import { Component } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-addition',
templateUrl: './addition.component.html',
styleUrl: './addition.component.css'
})
export class AdditionComponent {
data:number=0;
constructor(private activatedRoute: ActivatedRoute) {}
ngOnInit() {
// Subscribe to the route parameter changes
this.activatedRoute.params.subscribe(params => {
// Retrieve the 'id' parameter from the route
this.data = +params['id']; // Convert to a number if needed
});
}
}
write code on additioncomponent.html
{{data}}
Angular Form 17 Validation:-
First Install bootstrap
npm install bootstrap@4.6.2
add configuration under angular.json
"styles": [
"node_modules/bootstrap/dist/css/bootstrap.min.css",
"src/styles.css"
],
"scripts": [
"node_modules/jquery/dist/jquery.slim.min.js",
"node_modules/popper.js/dist/umd/popper.min.js",
"node_modules/bootstrap/dist/js/bootstrap.min.js"
]
Import Module under app.modul.ts file:-
import { FormsModule,ReactiveFormsModule} from '@angular/forms';
imports: [
FormsModule,
ReactiveFormsModule,
]
Step3rd:-
Create Component
Write following code on .ts file
import { Component } from '@angular/core';
import {ReactiveFormsModule} from '@angular/forms';
import {FormGroup, FormControl, Validators } from '@angular/forms';
@Component({
selector: 'app-formvaliation',
templateUrl: './formvaliation.component.html',
styleUrl: './formvaliation.component.css'
})
export class FormvaliationComponent {
form = new FormGroup({
name: new FormControl('', [Validators.required, Validators.minLength(3)]),
email: new FormControl('', [Validators.required, Validators.email]),
body: new FormControl('', Validators.required)
});
get f(){
return this.form.controls;
}
submit(){
console.log(this.form.value);
}
}
write following code in .html file
<p>formvaliation works!</p>
<form [formGroup]="form" (ngSubmit)="submit()">
<div class="form-group">
<label for="name">Name</label>
<input
formControlName="name"
id="name"
type="text"
class="form-control">
<div *ngIf="f['name'].touched && f['name'].invalid" class="alert alert-danger">
<div *ngIf="f['name'].errors && f['name'].errors['required']">Name is required.</div>
<div *ngIf="f['name'].errors && f['name'].errors['minlength']">Name should be 3 character.</div>
</div>
</div>
<div class="form-group">
<label for="email">Email</label>
<input
formControlName="email"
id="email"
type="text"
class="form-control">
<div *ngIf="f['email'].touched && f['email'].invalid" class="alert alert-danger">
<div *ngIf="f['email'].errors && f['email'].errors['required']">Email is required.</div>
<div *ngIf="f['email'].errors && f['email'].errors['email']">Please, enter valid email address.</div>
</div>
</div>
<div class="form-group">
<label for="body">Body</label>
<textarea
type="text"
formControlName="body"
id="body"
rows="5"
cols="50"
class="text-area"></textarea>
<div *ngIf="f['body'].touched && f['body'].invalid" class="alert alert-danger">
<div *ngIf="f['body'].errors && f['body'].errors['required']">Body is required.</div>
</div>
</div>
<button class="btn btn-primary" [disabled]="form.invalid" type="submit">Submit</button>
</form>
Comments
Post a Comment
POST Answer of Questions and ASK to Doubt